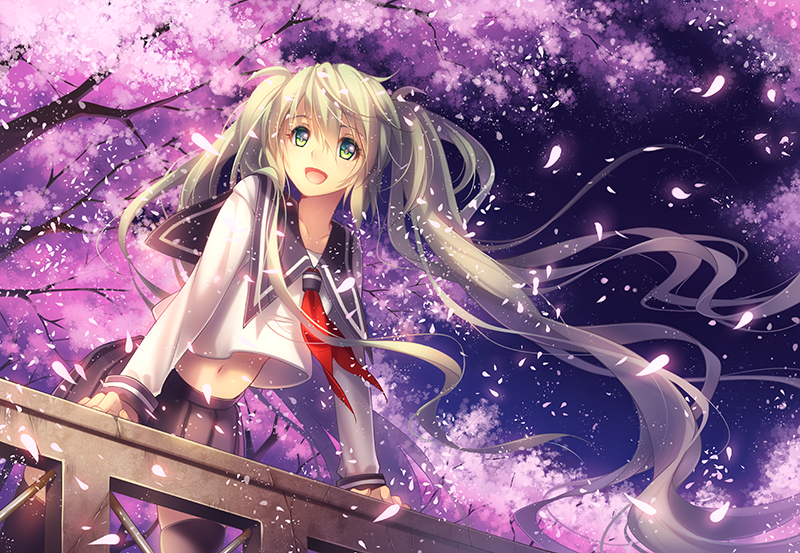
source: unknown
「東山覇権」盛行下的碧池这个词,几乎是个定番的萌点了。双马尾金发碧眼归国麻将日侨的威压统治了円光排行榜数载,
使得麻将军团的地位牢不可破。动画中一有搔首弄姿的镜头,屏幕前众宅男便心领神会。而年度nico某排行榜里,当壮大的BGM配着罗格翻开冠军ako酱的名字时,就能感到这王者风范:就像全屏大赤字弹幕一样霸气。
而在同人薄本界,霸主地位也高高在上遥不可及。《清纯奔放的木静酱》是一部不错的全彩短篇,可惜故事不成气候,难以动摇王座。然而,却有一部作品是円光界独树一帜的经典,震慑了女王新子瞳的王者地位——就是传说中感动2ch全J民的C79本《せめて、あの雪のように》。
这部作品是作者藤丸涉足同人界十周年的作品。虽说藤丸比较低调,并非是脍炙人口的名流。但画风却相当不错,线条俊秀,一本全彩本也相当实用,是个值得称道的绅士。
然而,这部十周年的作品,却并不是以其实用性著称,这正是她不同凡响之处。让这部作品不仅对作者而言纪念作品,更是援交作品界的一座里程碑式的存在。除此之外,同名小说以及MAD亦有爱好者制作,而作品的经典台词和分镜,更是渗入到了匿名基坛。可见《雪》是多么地震撼。
艺术手法上,《雪》是一部大量使用“留白”的作品,甚至可以说完全呈递了一张30页白纸给读者。与其浓墨重彩混体液描绘JK和大叔的床单故事,作品仅仅是让女主角雪和客人拉手让男主目击。余下的,就只是轻轻勾勒男女主间淡淡的青涩。这是一部“没有工口场景的工口作品”,没有一笔一墨淫猥,把故事和故事配套的动作戏码完全在读者脑内展开。也正是如此,原本是看中作者画风的看客,是硬生生把体液从下半身挤到了泪腺里流出来。
如果在这里做人物分析的话绝对属于多余,甚至是对作品的不敬。这部作品是经典,女主是经典的碧池妖精白无垢天使,男主是经典的迟钝缩卵。一个没有意外展开的故事,但确实是经典的故事,也正是一次、又一次碾碎读者心理防线的故事。前几页是Sucubus的开朗笑颜,下几页就是女主的无奈和夙愿。这笔触下的人物味道,不是死甜的糖也不是突兀的苦味,而是银勺轻舀咖啡,在口中化开甜味苦味醇香几个层次,在后味中品咖啡豆是如何烘焙烧制的——作品留白了女主雪的背景,却又提供了她的苦恼,让读者自然地在脑内补完。
因而刻画女主白山雪尤其经典,女主的每句台词都是经典
—— 好羡慕月村同学,真好啊,我也想谈恋爱
—— 花丘君对我没兴趣,所以喜欢,真的喜欢
—— 各种人,各种想法,我都懂,被教会了 (英译本中为 I was made to know真是被动语态在翻译暧昧语言的合适用法)
—— 像我这样的二手货,清仓甩卖也没人要啦
这似曾相识的句子,简直是掩藏在人类集体无意识中的原型,被挖掘出来压缩在几十页中冲击读者的精神。这,就是经典,恒久不变,历久弥新。
和众多薄本一样,《雪》足够给看客带来充分贤者时间。而不同的是,《雪》并不局限在短暂肤浅的生理不应期,而是对人性深层的震撼和拷问。在各处对援交的舌战),总是能看到在遥遥道德高点上用“干净”“肮脏”的概念,反对援交。在没有论证前就用着“试想蔚然成风后社会还能干净吗”的论调强占高点。然而《雪》这部作品却展现了另一面:少女在成长和生活中的无奈和困苦,迫使少女的早熟和世故。对援交的鄙夷在宏观上或许是大义凛然的,但在微观上却显得无情无义。或许不该忘记,在臆想中或污染社会的援交,恰恰是现实里由龌龊的社会所制造的。
当然,这部作品无法脱离校园恋爱剧的范畴,金钱交易和校园纯爱的反差也是看点之一,合乎心智(尤其异性人际方面)水平(滞留)在中学时代的宅男。而最后女主去向不明,成为男主的回忆,倒也是提供了不少可以意淫之处。这样的结局倒是影射了漫画的特色:任何角色,只能成为看客精神世界里的影子。《雪》确实挖了一道沟渠让读者能意淫出一道爱河了。
《せめて、あの雪のように》是一本让人心酸的本子。其影响已经超过作品本身,演化成了”像我这样的二手货,也不会要XXX”的成句,真是又给这个漫画添加了别样的风味。然而转念一想,可能更增加了一份实用。每每看到年轻情侣亲密时,总是会有难免见不得理还乱的忧愁;又或者看到大叔拉着百褶裙的手在草地上散步时,百感交集。然而重温这个经典的镜头和台词,却瞬间心头感到:这酸爽!
啊,这酸爽!至少、像那老坛酸菜牛肉面一样
作者简介:藤丸 漫画家,原画家, 曾用名F.blue,曾与ひらめ、綾瀬三人组团出本,不过其中一人在C75的时候撂了挑子(迷上了和洛奇很像的一种网游)。藤丸也就在那个时候改了名,自 己一个人单练。藤丸的作品不多,原创气息浓厚,双手磨砺的很充分,仅有的几部同人都有很高的还原度,分镜规矩,笔法公整,平凡的剧情,简直就是一个传统的 漫画家